This article is Part 5 in a 5-Part Series.
- Part 1 - Ubuntu-Zero To Deploy
- Part 2 - Z-shell
- Part 3 - Vim
- Part 4 - Cinnamon
- Part 5 - This Article
Rails is well-know web framework which is completely written in Ruby. I am not going to explain what Rails is and why you should start learning it..You can find many online resources to learn and master rails..
Learning Resources:
Installation
Rbenv
Rbenv is a simple Ruby version management tool which is similar to RVM..i prefer Rbenv over RVM because it is light and it uses shims to choose appropriate ruby versions.
# install dependencies
sudo apt-get update
sudo apt-get install git-core curl zlib1g-dev build-essential libssl-dev libreadline-dev libyaml-dev libsqlite3-dev sqlite3 libxml2-dev libxslt1-dev
# clone rbenv from github
git clone https://github.com/sstephenson/rbenv.git ~/.rbenv
# export path to zshrc or bashrc based on your shell
echo 'export PATH="$HOME/.rbenv/bin:$PATH"' >> ~/.zshrc
echo 'eval "$(rbenv init -)"' >> ~/.zshrc
exec $SHELL
git clone git://github.com/sstephenson/ruby-build.git ~/.rbenv/plugins/ruby-build
echo 'export PATH="$HOME/.rbenv/plugins/ruby-build/bin:$PATH"' >> ~/.zshrc
exec $SHELL
rbenv install 2.1.1
rbenv global 2.1.1
ruby -v
# Tell rubygems not to install documentation
echo "gem: --no-ri --no-rdoc" > ~/.gemrc
Configure Git
git config --global user.name "your user name"
git config --global user.email "mail@expamle.com"
git config --global color.ui true
# generate ssh key and add in github
ssh-keygen -t rsa -C "mail@example.com"
Go to GitHub settings page and paste the generated ssh and then check your ssh key..
` ssh -T git@github.com `
You should get a message like this:
Hi "your name" You've successfully authenticated, but GitHub does not provide shell access.
Install NodeJS
sudo add-apt-repository ppa:chris-lea/node.js
sudo apt-get update
sudo apt-get install nodejs
Install Rails
` gem install rails `
You should now tell rbenv to make the rails executable available.
` rbenv rehash `
Check rails version
rails -v
# Rails 4.0.2
Install Heroku
wget -qO- https://toolbelt.heroku.com/install-ubuntu.sh | sh
# login to heroku
heroku login
Enter your Heroku credentials.
Email: email@example.com
Password:
Authentication successful.
Lets create a sample Rails application and deploy in heroku using git..
rails new gitbook
create
create README.rdoc
create Rakefile
create config.ru
create .gitignore
create Gemfile
create app
create app/assets/javascripts/application.js
create app/assets/stylesheets/application.css
create app/controllers/application_controller.rb
create app/helpers/application_helper.rb
...
...
cd gitbook
git init
Initialized empty Git repository in /home/user/directory/gitbook/.git/
# add files to git
ga .
gcmsg "initial commit"
# add GitHub repo and push the files
git remote add origin git@github.com:username/gitbook.git
gp
Heroku uses PostgreSQL db and rails_12factor gem to serve static assets so lets open up our Gemfile and include those gems..
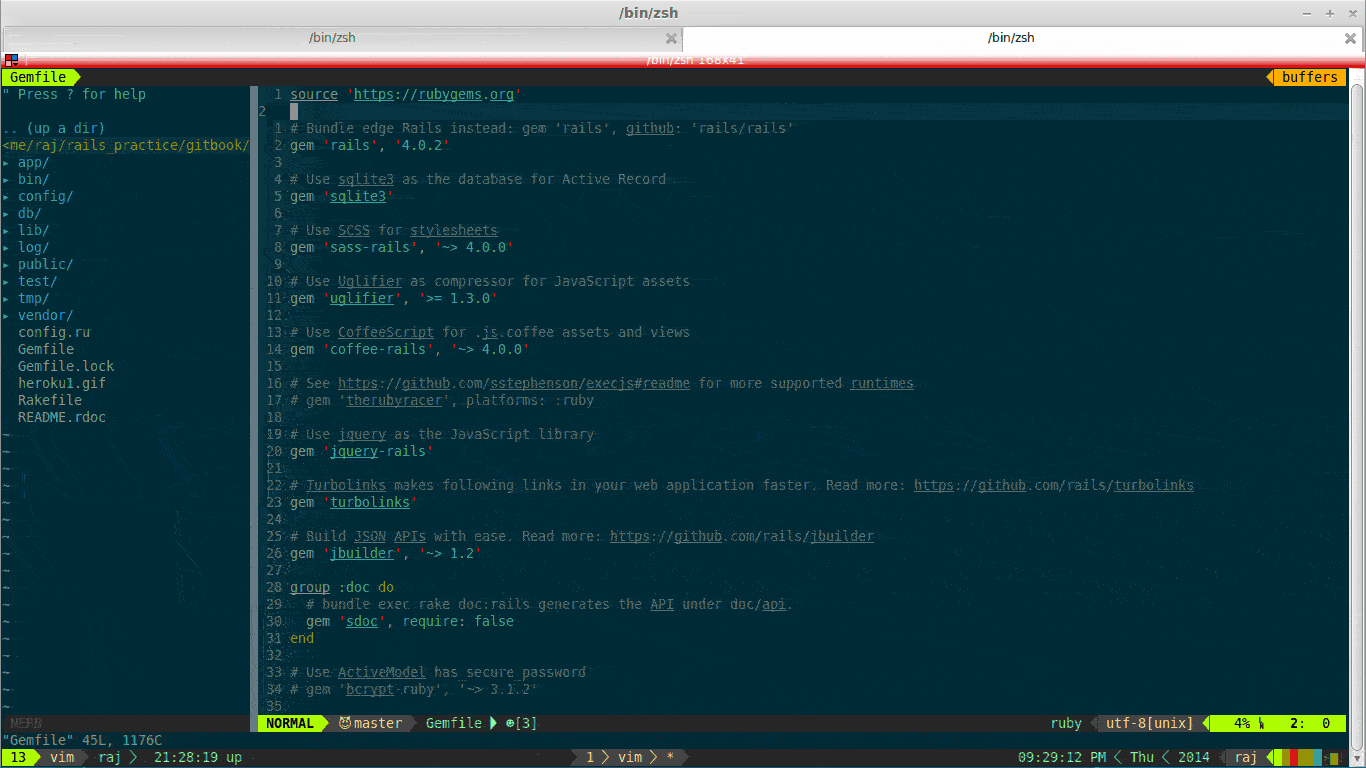
Commit those changes to git and deploy the app in heroku
ga Gemfile
gcmsg "include pg and rails_12factor for heroku deploy"
# create heroku application
heroku create
Creating infinite-shore-5163... done, stack is cedar
http://infinite-shore-5163.herokuapp.com/ | git@heroku.com:infinite-shore-5163.git
Git remote heroku added
# rename heroku app
heroku rename gitbookdemo (git)[master|… 53]
Renaming infinite-shore-5163 to gitbookdemo... done
http://gitbookdemo.herokuapp.com/ | git@heroku.com:gitbookdemo.git
Git remote heroku updated
# push the repo to Heroku
git push heroku master
Initializing repository, done.
Counting objects: 63, done.
Delta compression using up to 8 threads.
Compressing objects: 100% (52/52), done.
Writing objects: 100% (63/63), 14.57 KiB | 0 bytes/s, done.
Total 63 (delta 4), reused 0 (delta 0)
-----> Ruby app detected
-----> Compiling Ruby/Rails
-----> Using Ruby version: ruby-2.0.0
-----> Installing dependencies using 1.5.2
New app detected loading default bundler cache
Running: bundle install --without development:test --path vendor/bundle --binstubs vendor/bundle/bin -j4 --deployment
Fetching gem metadata from https://rubygems.org/.........
Fetching additional metadata from https://rubygems.org/..
Using rake (10.1.1)
Using multi_json (1.8.4)
...
...
-----> Compressing... done, 21.3MB
-----> Launching... done, v6
http://gitbookdemo.herokuapp.com/ deployed to Heroku
To git@heroku.com:gitbookdemo.git
* [new branch] master -> master
Open heroku app
` heroku open `
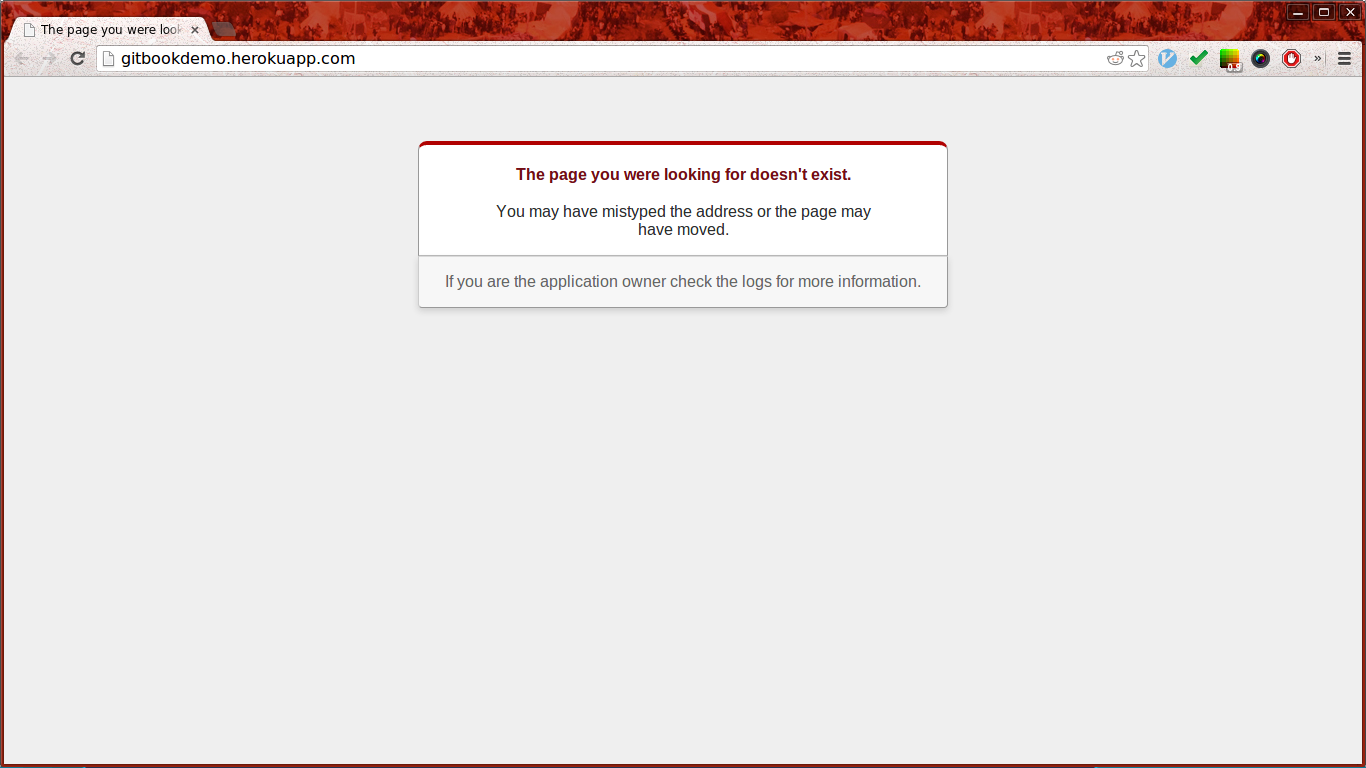
You would see an error page because after rails 4.0, for some technical reason heroku doesn’t show rails default home page but you could create your own page and deploy in heroku…
Wrap-up
We have successfully installed all the required packages and tool to make our distro ready for production..If you are not interested to do all these installations manually you should checkout Thoughbot’s laptop script that will install everything for you…
` bash <(wget -qO- https://raw.github.com/thoughtbot/laptop/master/linux)> `
You might also want to install the following tools for your every day usage ..